Audio addon
These functions are declared in the following header file. Link with allegro_audio.
#include <allegro5/allegro_audio.h>
Basic Audio
In order to just play some sounds (called samples in Allegro) and background music, here’s how to quick start with Allegro’s audio addon: Call al_reserve_samples with the number of samples you’d like to be able to play simultaneously (don’t forget to call al_install_audio beforehand). If these succeed, you can now call al_play_sample, with data obtained by al_load_sample, for example (don’t forget to initialize the acodec addon). In order to stop samples, you can use the ALLEGRO_SAMPLE_ID that al_play_sample returns.
If you want to play large audio files (e.g. background music) without
loading the whole file at once you can use al_play_audio_stream (after
calling al_reserve_samples).
This will load and play an ALLEGRO_AUDIO_STREAM
. Note that
the basic API only supports one such audio stream playing at once.
ALLEGRO_SAMPLE_ID
typedef struct ALLEGRO_SAMPLE_ID ALLEGRO_SAMPLE_ID;
An ALLEGRO_SAMPLE_ID represents a sample being played via al_play_sample. It can be used to later stop the sample with al_stop_sample. The underlying ALLEGRO_SAMPLE_INSTANCE can be extracted using al_lock_sample_id.
Examples:
al_install_audio
bool al_install_audio(void)
Install the audio subsystem.
Returns true on success, false on failure.
Note: most users will call al_reserve_samples and al_init_acodec_addon after this.
See also: al_reserve_samples, al_uninstall_audio, al_is_audio_installed, al_init_acodec_addon
Examples:
al_uninstall_audio
void al_uninstall_audio(void)
Uninstalls the audio subsystem.
See also: al_install_audio
Examples:
al_is_audio_installed
bool al_is_audio_installed(void)
Returns true if al_install_audio was called previously and returned successfully.
al_reserve_samples
bool al_reserve_samples(int reserve_samples)
Reserves a number of sample instances, attaching them to the default mixer. If no default mixer is set when this function is called, then it will create one and attach it to the default voice. If no default voice has been set, it, too, will be created.
If you call this function a second time with a smaller number of samples, then the excess internal sample instances will be destroyed causing some sounds to stop and some instances returned by al_lock_sample_id to be invalidated.
This diagram illustrates the structures that are set up:
sample instance 1
/ sample instance 2
default voice <-- default mixer <--- .
\ .
sample instance N
Returns true on success, false on error. al_install_audio must have been called first.
See also: al_set_default_mixer, al_play_sample
Examples:
al_play_sample
bool al_play_sample(ALLEGRO_SAMPLE *spl, float gain, float pan, float speed,
, ALLEGRO_SAMPLE_ID *ret_id) ALLEGRO_PLAYMODE loop
Plays a sample on one of the sample instances created by al_reserve_samples. Returns true on success, false on failure. Playback may fail because all the reserved sample instances are currently used.
Parameters:
- gain - relative volume at which the sample is played; 1.0 is normal.
- pan - 0.0 is centred, -1.0 is left, 1.0 is right, or ALLEGRO_AUDIO_PAN_NONE.
- speed - relative speed at which the sample is played; 1.0 is normal.
- loop - ALLEGRO_PLAYMODE_ONCE, ALLEGRO_PLAYMODE_LOOP, or ALLEGRO_PLAYMODE_BIDIR
- ret_id - if non-NULL the variable which this points to will be
assigned an id representing the sample being played. If al_play_sample returns
false
, then the contents of ret_id are invalid and must not be used as argument to other functions.
See also: al_load_sample, ALLEGRO_PLAYMODE, ALLEGRO_AUDIO_PAN_NONE, ALLEGRO_SAMPLE_ID, al_stop_sample, al_stop_samples, al_lock_sample_id.
Examples:
al_stop_sample
void al_stop_sample(ALLEGRO_SAMPLE_ID *spl_id)
Stop the sample started by al_play_sample.
See also: al_stop_samples
Examples:
al_stop_samples
void al_stop_samples(void)
Stop all samples started by al_play_sample.
See also: al_stop_sample
Examples:
al_lock_sample_id
* al_lock_sample_id(ALLEGRO_SAMPLE_ID *spl_id) ALLEGRO_SAMPLE_INSTANCE
Locks a ALLEGRO_SAMPLE_ID, returning the underlying ALLEGRO_SAMPLE_INSTANCE. This allows you to adjust the various properties of the instance (such as volume, pan, etc) while the sound is playing.
This function will return NULL
if the sound
corresponding to the id is no longer playing.
While locked, ALLEGRO_SAMPLE_ID
will be unavailable to
additional calls to al_play_sample, even if the sound
stops while locked. To put the ALLEGRO_SAMPLE_ID
back into
the pool for reuse, make sure to call al_unlock_sample_id
when you’re done with the instance.
See also: al_play_sample, al_unlock_sample_id
Since: 5.2.3
Unstable API: New API.
Examples:
al_unlock_sample_id
void al_unlock_sample_id(ALLEGRO_SAMPLE_ID *spl_id)
Unlocks a ALLEGRO_SAMPLE_ID, allowing future calls to al_play_sample to reuse it if possible. Note that after the id is unlocked, the ALLEGRO_SAMPLE_INSTANCE that was previously returned by al_lock_sample_id will possibly be playing a different sound, so you should only use it after locking the id again.
See also: al_play_sample, al_lock_sample_id
Since: 5.2.3
Unstable API: New API.
Examples:
al_play_audio_stream
*al_play_audio_stream(const char *filename) ALLEGRO_AUDIO_STREAM
Loads and plays an audio file, streaming from disk as it is needed. This API can only play one audio stream at a time. This requires a default mixer to be set, which is typically done via al_reserve_samples, but can also be done via al_set_default_mixer.
Returns the stream on success, NULL on failure. You must not destroy the returned stream, it will be automatically destroyed when the addon is shut down.
Note: the allegro_audio library does not support any audio file formats by default. You must use the allegro_acodec addon, or register your own format handler.
See also: al_play_audio_stream_f, al_load_audio_stream
Since: 5.2.8
Unstable API: New API.
Examples:
al_play_audio_stream_f
*al_play_audio_stream_f(ALLEGRO_FILE *fp, const char *ident) ALLEGRO_AUDIO_STREAM
Loads and plays an audio file from ALLEGRO_FILE stream, streaming it is needed. This API can only play one audio stream at a time. This requires a default mixer to be set, which is typically done via al_reserve_samples, but can also be done via al_set_default_mixer.
The file type is determined by the passed ‘ident’ parameter, which is a file name extension including the leading dot.
Returns the stream on success, NULL on failure. You must not destroy the returned stream, it will be automatically destroyed when the addon is shut down. On success the file should be considered owned by the audio stream, and will be closed when the audio stream is destroyed. On failure the file will be closed.
Note: the allegro_audio library does not support any audio file formats by default. You must use the allegro_acodec addon, or register your own format handler.
See also: al_play_audio_stream, al_load_audio_stream_f
Since: 5.2.8
Unstable API: New API.
Samples
ALLEGRO_SAMPLE
typedef struct ALLEGRO_SAMPLE ALLEGRO_SAMPLE;
An ALLEGRO_SAMPLE object stores the data necessary for playing pre-defined digital audio. It holds a user-specified PCM data buffer and information about its format (data length, depth, frequency, channel configuration). You can have the same ALLEGRO_SAMPLE playing multiple times simultaneously.
See also: ALLEGRO_SAMPLE_INSTANCE
Examples:
al_create_sample
*al_create_sample(void *buf, unsigned int samples,
ALLEGRO_SAMPLE unsigned int freq, ALLEGRO_AUDIO_DEPTH depth,
, bool free_buf) ALLEGRO_CHANNEL_CONF chan_conf
Create a sample data structure from the supplied buffer. If
free_buf
is true then the buffer will be freed with al_free when the sample data structure is
destroyed. For portability (especially Windows), the buffer should have
been allocated with al_malloc.
Otherwise you should free the sample data yourself.
A sample that is referred to by the samples
parameter
refers to a sequence channel intensities. E.g. if you’re making a stereo
sample with the samples
set to 4, then the layout of the
data in buf
will be:
LRLRLRLR
Where L and R are the intensities for the left and right channels respectively. A single sample, then, refers to the LR pair in this example.
To allocate a buffer of the correct size, you can use something like this:
int sample_size = al_get_channel_count(chan_conf)
* al_get_audio_depth_size(depth);
int bytes = samples * sample_size;
void *buffer = al_malloc(bytes);
See also: al_destroy_sample, ALLEGRO_AUDIO_DEPTH, ALLEGRO_CHANNEL_CONF
Examples:
al_load_sample
*al_load_sample(const char *filename) ALLEGRO_SAMPLE
Loads a few different audio file formats based on their extension.
Note that this stores the entire file in memory at once, which may be time consuming. To read the file as it is needed, use al_load_audio_stream or al_play_audio_stream.
Returns the sample on success, NULL on failure.
Note: the allegro_audio library does not support any audio file formats by default. You must use the allegro_acodec addon, or register your own format handler.
See also: al_register_sample_loader, al_init_acodec_addon
Examples:
al_load_sample_f
*al_load_sample_f(ALLEGRO_FILE* fp, const char *ident) ALLEGRO_SAMPLE
Loads an audio file from an ALLEGRO_FILE stream into an ALLEGRO_SAMPLE. The file type is determined by the passed ‘ident’ parameter, which is a file name extension including the leading dot.
Note that this stores the entire file in memory at once, which may be time consuming. To read the file as it is needed, use al_load_audio_stream_f or al_play_audio_stream_f.
Returns the sample on success, NULL on failure. The file remains open afterwards.
Note: the allegro_audio library does not support any audio file formats by default. You must use the allegro_acodec addon, or register your own format handler.
See also: al_register_sample_loader_f, al_init_acodec_addon
al_save_sample
bool al_save_sample(const char *filename, ALLEGRO_SAMPLE *spl)
Writes a sample into a file. Currently, wav is the only supported format, and the extension must be “.wav”.
Returns true on success, false on error.
Note: the allegro_audio library does not support any audio file formats by default. You must use the allegro_acodec addon, or register your own format handler.
See also: al_save_sample_f, al_register_sample_saver, al_init_acodec_addon
al_save_sample_f
bool al_save_sample_f(ALLEGRO_FILE *fp, const char *ident, ALLEGRO_SAMPLE *spl)
Writes a sample into a ALLEGRO_FILE filestream. Currently, wav is the only supported format, and the extension must be “.wav”.
Returns true on success, false on error. The file remains open afterwards.
Note: the allegro_audio library does not support any audio file formats by default. You must use the allegro_acodec addon, or register your own format handler.
See also: al_save_sample, al_register_sample_saver_f, al_init_acodec_addon
al_destroy_sample
void al_destroy_sample(ALLEGRO_SAMPLE *spl)
Free the sample data structure. If it was created with the
free_buf
parameter set to true, then the buffer will be
freed with al_free.
This function will stop any sample instances which may be playing the buffer referenced by the ALLEGRO_SAMPLE.
See also: al_destroy_sample_instance, al_stop_sample, al_stop_samples
Examples:
al_get_sample_channels
(const ALLEGRO_SAMPLE *spl) ALLEGRO_CHANNEL_CONF al_get_sample_channels
Return the channel configuration of the sample.
See also: ALLEGRO_CHANNEL_CONF, al_get_sample_depth, al_get_sample_frequency, al_get_sample_length, al_get_sample_data
al_get_sample_depth
(const ALLEGRO_SAMPLE *spl) ALLEGRO_AUDIO_DEPTH al_get_sample_depth
Return the audio depth of the sample.
See also: ALLEGRO_AUDIO_DEPTH, al_get_sample_channels, al_get_sample_frequency, al_get_sample_length, al_get_sample_data
al_get_sample_frequency
unsigned int al_get_sample_frequency(const ALLEGRO_SAMPLE *spl)
Return the frequency (in Hz) of the sample.
See also: al_get_sample_channels, al_get_sample_depth, al_get_sample_length, al_get_sample_data
al_get_sample_length
unsigned int al_get_sample_length(const ALLEGRO_SAMPLE *spl)
Return the length of the sample in sample values.
See also: al_get_sample_channels, al_get_sample_depth, al_get_sample_frequency, al_get_sample_data
al_get_sample_data
void *al_get_sample_data(const ALLEGRO_SAMPLE *spl)
Return a pointer to the raw sample data.
See also: al_get_sample_channels, al_get_sample_depth, al_get_sample_frequency, al_get_sample_length
Examples:
Advanced Audio
For more fine-grained control over audio output, here’s a short description of the basic concepts:
Voices represent audio devices on the system. Basically, every audio output chain that you want to be heard needs to end up in a voice. As voices are on the hardware/driver side of things, there is only limited control over their parameters (frequency, sample format, channel configuration). The number of available voices is limited as well. Typically, you will only use one voice and attach a mixer to it. Calling al_reserve_samples will do this for you by setting up a default voice and mixer; it can also be achieved by calling al_restore_default_mixer. Although you can attach sample instances and audio streams directly to a voice without using a mixer, it is, as of now, not recommended. In contrast to mixers, you can only attach a single object to a voice anyway.
Mixers mix several sample instances and/or audio streams into a single output buffer, converting sample data with differing formats according to their output parameters (frequency, depth, channels) in the process. In order to play several samples/streams at once reliably, you will need at least one mixer. A mixer that is not (indirectly) attached to a voice will remain silent. For most use cases, one (default) mixer attached to a single voice will be sufficient. You may attach mixers to other mixers in order to create complex audio chains.
Samples (ALLEGRO_SAMPLE) just represent “passive” buffers for sample data in memory. In order to play a sample, a sample instance (ALLEGRO_SAMPLE_INSTANCE) needs to be created and attached to a mixer (or voice). Sample instances control how the underlying samples are played. Several playback parameters (position, speed, gain, pan, playmode, playing/paused) can be adjusted. Particularly, multiple instances may be created from the same sample, e.g. with different parameters.
Audio streams (ALLEGRO_AUDIO_STREAM) are similar to sample instances insofar as they respond to the same playback parameters and have to be attached to mixers or voices. A single audio stream can only be played once simultaneously.
For example, consider the following configuration of the audio system.
* voice = al_create_voice(44100, ALLEGRO_AUDIO_DEPTH_INT16,
ALLEGRO_VOICE);
ALLEGRO_CHANNEL_CONF_2
* mixer_1 = al_create_mixer(44100, ALLEGRO_AUDIO_DEPTH_FLOAT32,
ALLEGRO_MIXER);
ALLEGRO_CHANNEL_CONF_2* mixer_2 = al_create_mixer(44100, ALLEGRO_AUDIO_DEPTH_FLOAT32,
ALLEGRO_MIXER);
ALLEGRO_CHANNEL_CONF_2
/* Load a stream, the stream starts in a playing state and just needs
* to be attached to actually output sound. */
* stream = al_load_audio_stream("music.ogg", 4, 2048);
ALLEGRO_AUDIO_STREAM
/* The sample needs sample instances to output sound. */
* sample = al_load_sample("sound.wav")
ALLEGRO_SAMPLE* instance_1 = al_create_sample_instance(sample);
ALLEGRO_SAMPLE_INSTANCE* instance_2 = al_create_sample_instance(sample);
ALLEGRO_SAMPLE_INSTANCE
/* Attach everything up (see diagram). */
(mixer_1, voice);
al_attach_mixer_to_voice(mixer_2, mixer_1);
al_attach_mixer_to_mixer(stream, mixer_1);
al_attach_audio_stream_to_mixer(instance_1, mixer_2);
al_attach_sample_instance_to_mixer(instance_2, mixer_2);
al_attach_sample_instance_to_mixer
/* Play two copies of the sound simultaneously. */
(instance_1, true);
al_set_sample_instance_playing(instance_2, true); al_set_sample_instance_playing
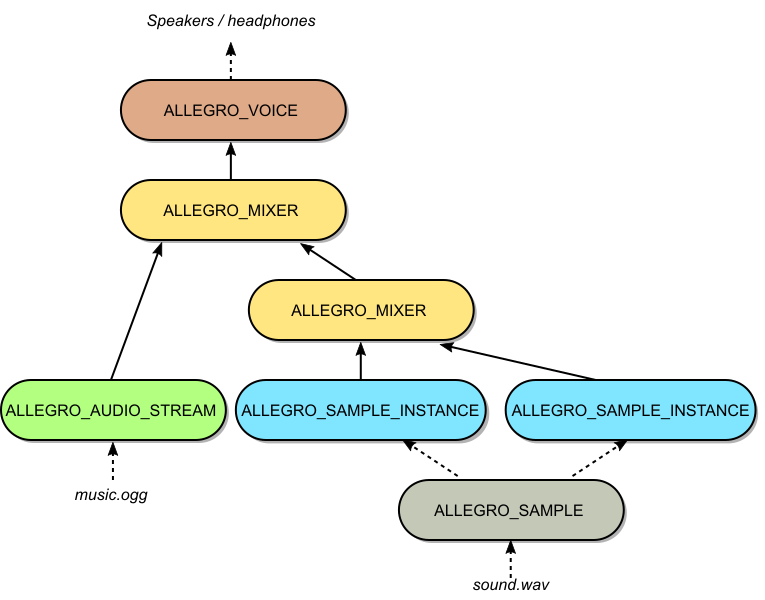
Since we have two mixers, with the sample instances connected to a different mixer than the audio stream, you can control the volume of all the instances independently from the music by setting the gain of the mixer / stream. Having two sample instances lets you play two copies of the sample simultaneously.
With this in mind, another look at al_reserve_samples and al_play_sample is due: What the former does internally is to create a specified number of sample instances that are “empty” at first, i.e. with no sample data set. When al_play_sample is called, it’ll use one of these internal sample instances that is not currently playing to play the requested sample. All of these sample instances will be attached to the default mixer, which can be changed via al_set_default_mixer.
Sample instances
ALLEGRO_SAMPLE_INSTANCE
typedef struct ALLEGRO_SAMPLE_INSTANCE ALLEGRO_SAMPLE_INSTANCE;
An ALLEGRO_SAMPLE_INSTANCE object represents a playable instance of a predefined sound effect. It holds information about how the effect should be played: These playback parameters consist of the looping mode, loop start/end points, playing position, speed, gain, pan and the playmode. Whether a sample instance is currently playing or paused is also one of its properties.
An instance uses the data from an ALLEGRO_SAMPLE object. Multiple instances may be created from the same ALLEGRO_SAMPLE. An ALLEGRO_SAMPLE must not be destroyed while there are instances which reference it.
To actually produce audio output, an ALLEGRO_SAMPLE_INSTANCE must be attached to an ALLEGRO_MIXER which eventually reaches an ALLEGRO_VOICE object.
See also: ALLEGRO_SAMPLE
Examples:
al_create_sample_instance
*al_create_sample_instance(ALLEGRO_SAMPLE *sample_data) ALLEGRO_SAMPLE_INSTANCE
Creates a sample instance, using the supplied sample data. The instance must be attached to a mixer (or voice) in order to actually produce output.
The argument may be NULL. You can then set the sample data later with al_set_sample.
See also: al_destroy_sample_instance
Examples:
al_destroy_sample_instance
void al_destroy_sample_instance(ALLEGRO_SAMPLE_INSTANCE *spl)
Detaches the sample instance from anything it may be attached to and frees it (the sample data, i.e. its ALLEGRO_SAMPLE, is not freed!).
See also: al_create_sample_instance
Examples:
al_play_sample_instance
bool al_play_sample_instance(ALLEGRO_SAMPLE_INSTANCE *spl)
Play the sample instance. Returns true on success, false on failure.
See also: al_stop_sample_instance
Examples:
al_stop_sample_instance
bool al_stop_sample_instance(ALLEGRO_SAMPLE_INSTANCE *spl)
Stop an sample instance playing.
See also: al_play_sample_instance
Examples:
al_get_sample_instance_channels
(
ALLEGRO_CHANNEL_CONF al_get_sample_instance_channelsconst ALLEGRO_SAMPLE_INSTANCE *spl)
Return the channel configuration of the sample instance’s sample data.
See also: ALLEGRO_CHANNEL_CONF.
Examples:
al_get_sample_instance_depth
(const ALLEGRO_SAMPLE_INSTANCE *spl) ALLEGRO_AUDIO_DEPTH al_get_sample_instance_depth
Return the audio depth of the sample instance’s sample data.
See also: ALLEGRO_AUDIO_DEPTH.
Examples:
al_get_sample_instance_frequency
unsigned int al_get_sample_instance_frequency(const ALLEGRO_SAMPLE_INSTANCE *spl)
Return the frequency (in Hz) of the sample instance’s sample data.
Examples:
al_get_sample_instance_length
unsigned int al_get_sample_instance_length(const ALLEGRO_SAMPLE_INSTANCE *spl)
Return the length of the sample instance in sample values. This property may differ from the length of the instance’s sample data.
See also: al_set_sample_instance_length, al_get_sample_instance_time
Examples:
al_set_sample_instance_length
bool al_set_sample_instance_length(ALLEGRO_SAMPLE_INSTANCE *spl,
unsigned int val)
Set the length of the sample instance in sample values. This can be used to play only parts of the underlying sample. Be careful not to exceed the actual length of the sample data, though.
Return true on success, false on failure. Will fail if the sample instance is currently playing.
See also: al_get_sample_instance_length
Examples:
al_get_sample_instance_position
unsigned int al_get_sample_instance_position(const ALLEGRO_SAMPLE_INSTANCE *spl)
Get the playback position of a sample instance.
See also: al_set_sample_instance_position
Examples:
al_set_sample_instance_position
bool al_set_sample_instance_position(ALLEGRO_SAMPLE_INSTANCE *spl,
unsigned int val)
Set the playback position of a sample instance.
Returns true on success, false on failure.
See also: al_get_sample_instance_position
Examples:
al_get_sample_instance_speed
float al_get_sample_instance_speed(const ALLEGRO_SAMPLE_INSTANCE *spl)
Return the relative playback speed of the sample instance.
See also: al_set_sample_instance_speed
al_set_sample_instance_speed
bool al_set_sample_instance_speed(ALLEGRO_SAMPLE_INSTANCE *spl, float val)
Set the relative playback speed of the sample instance. 1.0 means normal speed.
Return true on success, false on failure. Will fail if the sample instance is attached directly to a voice.
See also: al_get_sample_instance_speed
Examples:
al_get_sample_instance_gain
float al_get_sample_instance_gain(const ALLEGRO_SAMPLE_INSTANCE *spl)
Return the playback gain of the sample instance.
See also: al_set_sample_instance_gain
Examples:
al_set_sample_instance_gain
bool al_set_sample_instance_gain(ALLEGRO_SAMPLE_INSTANCE *spl, float val)
Set the playback gain of the sample instance.
Returns true on success, false on failure. Will fail if the sample instance is attached directly to a voice.
See also: al_get_sample_instance_gain
Examples:
al_get_sample_instance_pan
float al_get_sample_instance_pan(const ALLEGRO_SAMPLE_INSTANCE *spl)
Get the pan value of the sample instance.
See also: al_set_sample_instance_pan.
al_set_sample_instance_pan
bool al_set_sample_instance_pan(ALLEGRO_SAMPLE_INSTANCE *spl, float val)
Set the pan value on a sample instance. A value of -1.0 means to play the sample only through the left speaker; +1.0 means only through the right speaker; 0.0 means the sample is centre balanced. A special value ALLEGRO_AUDIO_PAN_NONE disables panning and plays the sample at its original level. This will be louder than a pan value of 0.0.
Note: panning samples with more than two channels doesn’t work yet.
Returns true on success, false on failure. Will fail if the sample instance is attached directly to a voice.
See also: al_get_sample_instance_pan, ALLEGRO_AUDIO_PAN_NONE
Examples:
al_get_sample_instance_time
float al_get_sample_instance_time(const ALLEGRO_SAMPLE_INSTANCE *spl)
Return the length of the sample instance in seconds, assuming a playback speed of 1.0.
See also: al_get_sample_instance_length
Examples:
al_get_sample_instance_playmode
(const ALLEGRO_SAMPLE_INSTANCE *spl) ALLEGRO_PLAYMODE al_get_sample_instance_playmode
Return the playback mode of the sample instance.
See also: ALLEGRO_PLAYMODE, al_set_sample_instance_playmode
al_set_sample_instance_playmode
bool al_set_sample_instance_playmode(ALLEGRO_SAMPLE_INSTANCE *spl,
) ALLEGRO_PLAYMODE val
Set the playback mode of the sample instance.
Returns true on success, false on failure.
See also: ALLEGRO_PLAYMODE, al_get_sample_instance_playmode
Examples:
al_get_sample_instance_playing
bool al_get_sample_instance_playing(const ALLEGRO_SAMPLE_INSTANCE *spl)
Return true if the sample instance is in the playing state. This may be true even if the instance is not attached to anything.
See also: al_set_sample_instance_playing
Examples:
al_set_sample_instance_playing
bool al_set_sample_instance_playing(ALLEGRO_SAMPLE_INSTANCE *spl, bool val)
Change whether the sample instance is playing.
The instance does not need to be attached to anything (since: 5.1.8).
Returns true on success, false on failure.
See also: al_get_sample_instance_playing
Examples:
al_get_sample_instance_attached
bool al_get_sample_instance_attached(const ALLEGRO_SAMPLE_INSTANCE *spl)
Return whether the sample instance is attached to something.
See also: al_attach_sample_instance_to_mixer, al_attach_sample_instance_to_voice, al_detach_sample_instance
al_detach_sample_instance
bool al_detach_sample_instance(ALLEGRO_SAMPLE_INSTANCE *spl)
Detach the sample instance from whatever it’s attached to, if anything.
Returns true on success.
See also: al_attach_sample_instance_to_mixer, al_attach_sample_instance_to_voice, al_get_sample_instance_attached
Examples:
al_get_sample
*al_get_sample(ALLEGRO_SAMPLE_INSTANCE *spl) ALLEGRO_SAMPLE
Return the sample data that the sample instance plays.
Note this returns a pointer to an internal structure, not the ALLEGRO_SAMPLE that you may have passed to al_set_sample. However, the sample buffer of the returned ALLEGRO_SAMPLE will be the same as the one that was used to create the sample (passed to al_create_sample). You can use al_get_sample_data on the return value to retrieve and compare it.
See also: al_set_sample
Examples:
al_set_sample
bool al_set_sample(ALLEGRO_SAMPLE_INSTANCE *spl, ALLEGRO_SAMPLE *data)
Change the sample data that a sample instance plays. This can be quite an involved process.
First, the sample is stopped if it is not already.
Next, if data is NULL, the sample is detached from its parent (if any).
If data is not NULL, the sample may be detached and reattached to its parent (if any). This is not necessary if the old sample data and new sample data have the same frequency, depth and channel configuration. Reattaching may not always succeed.
On success, the sample remains stopped. The playback position and loop end points are reset to their default values. The loop mode remains unchanged.
Returns true on success, false on failure. On failure, the sample will be stopped and detached from its parent.
See also: al_get_sample
Examples:
al_set_sample_instance_channel_matrix
bool al_set_sample_instance_channel_matrix(ALLEGRO_SAMPLE_INSTANCE *spl, const float *matrix)
Set the matrix used to mix the channels coming from this instance into the mixer it is attached to. Normally Allegro derives the values of this matrix from the gain and pan settings, as well as the channel configurations of this instance and the mixer it is attached to, but this allows you override that default value. Note that if you do set gain or pan of this instance or the mixer it is attached to, you’ll need to call this function again.
The matrix has mixer channel rows and sample channel columns, and is row major. For example, if you have a stereo sample instance and want to mix it to a 5.1 mixer you could use this code:
float matrix[] = {
0.5, 0.0, /* Half left to front left */
0.0, 0.5, /* Half right to front right */
0.5, 0.0, /* Half left to rear left */
0.0, 0.5, /* Half right to rear right */
0.1, 0.1, /* Mix left and right for center */
0.1, 0.1, /* Mix left and right for center */
};
(instance, matrix); al_set_sample_instance_channel_matrix
Returns true on success, false on failure (e.g. if this is not attached to a mixer).
Since: 5.2.3
Unstable API: New API.
Examples:
Audio streams
ALLEGRO_AUDIO_STREAM
typedef struct ALLEGRO_AUDIO_STREAM ALLEGRO_AUDIO_STREAM;
An ALLEGRO_AUDIO_STREAM object is used to stream generated audio to the sound device, in real-time. This is done by reading from a buffer, which is split into a number of fragments. Whenever a fragment has finished playing, the user can refill it with new data.
As with ALLEGRO_SAMPLE_INSTANCE objects, streams store information necessary for playback, so you may not play the same stream multiple times simultaneously. Streams also need to be attached to an ALLEGRO_MIXER, which, eventually, reaches an ALLEGRO_VOICE object.
While playing, you must periodically fill fragments with new audio data. To know when a new fragment is ready to be filled, you can either directly check with al_get_available_audio_stream_fragments, or listen to events from the stream.
You can register an audio stream event source to an event queue; see al_get_audio_stream_event_source. An ALLEGRO_EVENT_AUDIO_STREAM_FRAGMENT event is generated whenever a new fragment is ready. When you receive an event, use al_get_audio_stream_fragment to obtain a pointer to the fragment to be filled. The size and format are determined by the parameters passed to al_create_audio_stream.
If you’re late with supplying new data, the stream will be silent until new data is provided. You must call al_drain_audio_stream when you’re finished with supplying data to the stream.
It is often a good idea to prefill the audio stream with data before ALLEGRO_EVENT_AUDIO_STREAM_FRAGMENT events arrive. Here is a snippet that will fill the stream buffers with silence:
*stream = al_create_audio_stream(num_buffers,
ALLEGRO_AUDIO_STREAM , freq, depth, channel_conf);
samples_per_buffervoid *buf;
while ((buf = al_get_audio_stream_fragment(stream))) {
(buf, samples_per_buffer, depth, channel_conf);
al_fill_silence(stream, buf);
al_set_audio_stream_fragment}
If the stream is created by al_load_audio_stream or al_play_audio_stream then it will also generate an ALLEGRO_EVENT_AUDIO_STREAM_FINISHED event if it reaches the end of the file and is not set to loop.
Examples:
al_create_audio_stream
*al_create_audio_stream(size_t fragment_count,
ALLEGRO_AUDIO_STREAM unsigned int frag_samples, unsigned int freq, ALLEGRO_AUDIO_DEPTH depth,
) ALLEGRO_CHANNEL_CONF chan_conf
Creates an ALLEGRO_AUDIO_STREAM. The stream will be set to play by default. It will feed audio data from a buffer, which is split into a number of fragments.
Parameters:
fragment_count - How many fragments to use for the audio stream. Usually only two fragments are required - splitting the audio buffer in two halves. But it means that the only time when new data can be supplied is whenever one half has finished playing. When using many fragments, you usually will use fewer samples for one, so there always will be (small) fragments available to be filled with new data.
frag_samples - The size of a fragment in samples. See note and explanation below.
freq - The frequency, in Hertz.
depth - Must be one of the values listed for ALLEGRO_AUDIO_DEPTH.
chan_conf - Must be one of the values listed for ALLEGRO_CHANNEL_CONF.
A sample that is referred to by the frag_samples parameter refers to a sequence channel intensities. E.g. if you’re making a stereo stream with the frag_samples set to 4, then the layout of the data in the fragment will be:
LRLRLRLR
Where L and R are the intensities for the left and right channels respectively. A single sample, then, refers to the LR pair in this example.
The choice of fragment_count, frag_samples and freq directly influences the audio delay. The delay in seconds can be expressed as:
delay = fragment_count * frag_samples / freq
This is only the delay due to Allegro’s streaming, there may be additional delay caused by sound drivers and/or hardware.
Note: If you know the fragment size in bytes, you can get the size in samples like this:
sample_size = al_get_channel_count(chan_conf) * al_get_audio_depth_size(depth); samples = bytes_per_fragment / sample_size;
The size of the complete buffer is:
buffer_size = bytes_per_fragment * fragment_count
Note: Unlike many Allegro objects, audio streams are not implicitly destroyed when Allegro is shut down. You must destroy them manually with al_destroy_audio_stream before the audio system is shut down.
Examples:
al_load_audio_stream
*al_load_audio_stream(const char *filename,
ALLEGRO_AUDIO_STREAM size_t buffer_count, unsigned int samples)
Loads an audio file from disk as it is needed.
Unlike regular streams, the one returned by this function need not be fed by the user; the library will automatically read more of the file as it is needed. The stream will contain buffer_count buffers with samples samples.
The audio stream will start in the playing state. It should be attached to a voice or mixer to generate any output. See ALLEGRO_AUDIO_STREAM for more details.
Returns the stream on success, NULL on failure.
Note: the allegro_audio library does not support any audio file formats by default. You must use the allegro_acodec addon, or register your own format handler.
See also: al_load_audio_stream_f, al_register_audio_stream_loader, al_init_acodec_addon
Examples:
al_load_audio_stream_f
*al_load_audio_stream_f(ALLEGRO_FILE* fp, const char *ident,
ALLEGRO_AUDIO_STREAM size_t buffer_count, unsigned int samples)
Loads an audio file from ALLEGRO_FILE stream as it is needed.
Unlike regular streams, the one returned by this function need not be fed by the user; the library will automatically read more of the file as it is needed. The stream will contain buffer_count buffers with samples samples.
The file type is determined by the passed ‘ident’ parameter, which is a file name extension including the leading dot.
The audio stream will start in the playing state. It should be attached to a voice or mixer to generate any output. See ALLEGRO_AUDIO_STREAM for more details.
Returns the stream on success, NULL on failure. On success the file should be considered owned by the audio stream, and will be closed when the audio stream is destroyed. On failure the file will be closed.
Note: the allegro_audio library does not support any audio file formats by default. You must use the allegro_acodec addon, or register your own format handler.
See also: al_load_audio_stream, al_register_audio_stream_loader_f, al_init_acodec_addon
al_destroy_audio_stream
void al_destroy_audio_stream(ALLEGRO_AUDIO_STREAM *stream)
Destroy an audio stream which was created with al_create_audio_stream or al_load_audio_stream.
Note: If the stream is still attached to a mixer or voice, al_detach_audio_stream is automatically called on it first.
See also: al_drain_audio_stream.
Examples:
al_get_audio_stream_event_source
*al_get_audio_stream_event_source(
ALLEGRO_EVENT_SOURCE *stream) ALLEGRO_AUDIO_STREAM
Retrieve the associated event source.
See al_get_audio_stream_fragment for a description of the ALLEGRO_EVENT_AUDIO_STREAM_FRAGMENT event that audio streams emit.
Examples:
al_drain_audio_stream
void al_drain_audio_stream(ALLEGRO_AUDIO_STREAM *stream)
You should call this to finalise an audio stream that you will no longer be feeding, to wait for all pending buffers to finish playing. The stream’s playing state will change to false.
See also: al_destroy_audio_stream
Examples:
al_rewind_audio_stream
bool al_rewind_audio_stream(ALLEGRO_AUDIO_STREAM *stream)
Set the streaming file playing position to the beginning. Returns true on success. Currently this can only be called on streams created with al_load_audio_stream, al_play_audio_stream, al_load_audio_stream_f or al_play_audio_stream_f.
Examples:
al_get_audio_stream_frequency
unsigned int al_get_audio_stream_frequency(const ALLEGRO_AUDIO_STREAM *stream)
Return the stream frequency (in Hz).
al_get_audio_stream_channels
(
ALLEGRO_CHANNEL_CONF al_get_audio_stream_channelsconst ALLEGRO_AUDIO_STREAM *stream)
Return the stream channel configuration.
See also: ALLEGRO_CHANNEL_CONF.
al_get_audio_stream_depth
(
ALLEGRO_AUDIO_DEPTH al_get_audio_stream_depthconst ALLEGRO_AUDIO_STREAM *stream)
Return the stream audio depth.
See also: ALLEGRO_AUDIO_DEPTH.
al_get_audio_stream_length
unsigned int al_get_audio_stream_length(const ALLEGRO_AUDIO_STREAM *stream)
Return the stream length in samples.
Examples:
al_get_audio_stream_speed
float al_get_audio_stream_speed(const ALLEGRO_AUDIO_STREAM *stream)
Return the relative playback speed of the stream.
See also: al_set_audio_stream_speed.
al_set_audio_stream_speed
bool al_set_audio_stream_speed(ALLEGRO_AUDIO_STREAM *stream, float val)
Set the relative playback speed of the stream. 1.0 means normal speed.
Return true on success, false on failure. Will fail if the audio stream is attached directly to a voice.
See also: al_get_audio_stream_speed.
al_get_audio_stream_gain
float al_get_audio_stream_gain(const ALLEGRO_AUDIO_STREAM *stream)
Return the playback gain of the stream.
See also: al_set_audio_stream_gain.
Examples:
al_set_audio_stream_gain
bool al_set_audio_stream_gain(ALLEGRO_AUDIO_STREAM *stream, float val)
Set the playback gain of the stream.
Returns true on success, false on failure. Will fail if the audio stream is attached directly to a voice.
See also: al_get_audio_stream_gain.
Examples:
al_get_audio_stream_pan
float al_get_audio_stream_pan(const ALLEGRO_AUDIO_STREAM *stream)
Get the pan value of the stream.
See also: al_set_audio_stream_pan.
al_set_audio_stream_pan
bool al_set_audio_stream_pan(ALLEGRO_AUDIO_STREAM *stream, float val)
Set the pan value on an audio stream. A value of -1.0 means to play the stream only through the left speaker; +1.0 means only through the right speaker; 0.0 means the sample is centre balanced. A special value ALLEGRO_AUDIO_PAN_NONE disables panning and plays the stream at its original level. This will be louder than a pan value of 0.0.
Returns true on success, false on failure. Will fail if the audio stream is attached directly to a voice.
See also: al_get_audio_stream_pan, ALLEGRO_AUDIO_PAN_NONE
Examples:
al_get_audio_stream_playing
bool al_get_audio_stream_playing(const ALLEGRO_AUDIO_STREAM *stream)
Return true if the stream is playing.
See also: al_set_audio_stream_playing.
Examples:
al_set_audio_stream_playing
bool al_set_audio_stream_playing(ALLEGRO_AUDIO_STREAM *stream, bool val)
Change whether the stream is playing.
Returns true on success, false on failure.
See also: al_get_audio_stream_playing
Examples:
al_get_audio_stream_playmode
(
ALLEGRO_PLAYMODE al_get_audio_stream_playmodeconst ALLEGRO_AUDIO_STREAM *stream)
Return the playback mode of the stream.
See also: ALLEGRO_PLAYMODE, al_set_audio_stream_playmode.
al_set_audio_stream_playmode
bool al_set_audio_stream_playmode(ALLEGRO_AUDIO_STREAM *stream,
) ALLEGRO_PLAYMODE val
Set the playback mode of the stream.
Returns true on success, false on failure.
See also: ALLEGRO_PLAYMODE, al_get_audio_stream_playmode.
Examples:
al_get_audio_stream_attached
bool al_get_audio_stream_attached(const ALLEGRO_AUDIO_STREAM *stream)
Return whether the stream is attached to something.
See also: al_attach_audio_stream_to_mixer, al_attach_audio_stream_to_voice, al_detach_audio_stream.
al_detach_audio_stream
bool al_detach_audio_stream(ALLEGRO_AUDIO_STREAM *stream)
Detach the stream from whatever it’s attached to, if anything.
See also: al_attach_audio_stream_to_mixer, al_attach_audio_stream_to_voice, al_get_audio_stream_attached.
Examples:
al_get_audio_stream_played_samples
uint64_t al_get_audio_stream_played_samples(const ALLEGRO_AUDIO_STREAM *stream)
Get the number of samples consumed by the parent since the audio stream was started.
Since: 5.1.8
al_get_audio_stream_fragment
void *al_get_audio_stream_fragment(const ALLEGRO_AUDIO_STREAM *stream)
When using Allegro’s audio streaming, you will use this function to continuously provide new sample data to a stream.
If the stream is ready for new data, the function will return the address of an internal buffer to be filled with audio data. The length and format of the buffer are specified with al_create_audio_stream or can be queried with the various functions described here. Once the buffer is filled, you must signal this to Allegro by passing the buffer to al_set_audio_stream_fragment.
If the stream is not ready for new data, the function will return NULL.
Note: If you listen to events from the stream, an ALLEGRO_EVENT_AUDIO_STREAM_FRAGMENT event will be generated whenever a new fragment is ready. However, getting an event is not a guarantee that al_get_audio_stream_fragment will not return NULL, so you still must check for it.
See also: al_set_audio_stream_fragment, al_get_audio_stream_event_source, al_get_audio_stream_frequency, al_get_audio_stream_channels, al_get_audio_stream_depth, al_get_audio_stream_length
Examples:
al_set_audio_stream_fragment
bool al_set_audio_stream_fragment(ALLEGRO_AUDIO_STREAM *stream, void *val)
This function needs to be called for every successful call of al_get_audio_stream_fragment
to indicate that the buffer (pointed to by val
) is filled
with new data.
See also: al_get_audio_stream_fragment
Examples:
al_get_audio_stream_fragments
unsigned int al_get_audio_stream_fragments(const ALLEGRO_AUDIO_STREAM *stream)
Returns the number of fragments this stream uses. This is the same value as passed to al_create_audio_stream when a new stream is created.
See also: al_get_available_audio_stream_fragments
al_get_available_audio_stream_fragments
unsigned int al_get_available_audio_stream_fragments(
const ALLEGRO_AUDIO_STREAM *stream)
Returns the number of available fragments in the stream, that is, fragments which are not currently filled with data for playback.
See also: al_get_audio_stream_fragment, al_get_audio_stream_fragments
al_seek_audio_stream_secs
bool al_seek_audio_stream_secs(ALLEGRO_AUDIO_STREAM *stream, double time)
Set the streaming file playing position to time. Returns true on success. Currently this can only be called on streams created with al_load_audio_stream, al_play_audio_stream, al_load_audio_stream_f or al_play_audio_stream_f.
See also: al_get_audio_stream_position_secs, al_get_audio_stream_length_secs
Examples:
al_get_audio_stream_position_secs
double al_get_audio_stream_position_secs(ALLEGRO_AUDIO_STREAM *stream)
Return the position of the stream in seconds. Currently this can only be called on streams created with al_load_audio_stream, al_play_audio_stream, al_load_audio_stream_f or al_play_audio_stream_f.
See also: al_get_audio_stream_length_secs
Examples:
al_get_audio_stream_length_secs
double al_get_audio_stream_length_secs(ALLEGRO_AUDIO_STREAM *stream)
Return the length of the stream in seconds, if known. Otherwise returns zero.
Currently this can only be called on streams created with al_load_audio_stream, al_play_audio_stream, al_load_audio_stream_f or al_play_audio_stream_f.
See also: al_get_audio_stream_position_secs
Examples:
al_set_audio_stream_loop_secs
bool al_set_audio_stream_loop_secs(ALLEGRO_AUDIO_STREAM *stream,
double start, double end)
Sets the loop points for the stream in seconds. Currently this can only be called on streams created with al_load_audio_stream, al_play_audio_stream, al_load_audio_stream_f or al_play_audio_stream_f.
Examples:
al_set_audio_stream_channel_matrix
Like al_set_sample_instance_channel_matrix but for streams.
Since: 5.2.3
Unstable API: New API.
Advanced audio file I/O
al_register_sample_loader
bool al_register_sample_loader(const char *ext,
*(*loader)(const char *filename)) ALLEGRO_SAMPLE
Register a handler for al_load_sample. The given function will be used to handle the loading of sample files with the given extension.
The extension should include the leading dot (‘.’) character. It will be matched case-insensitively.
The loader
argument may be NULL to unregister an
entry.
Returns true on success, false on error. Returns false if unregistering an entry that doesn’t exist.
See also: al_register_sample_loader_f, al_register_sample_saver
al_register_sample_loader_f
bool al_register_sample_loader_f(const char *ext,
*(*loader)(ALLEGRO_FILE* fp)) ALLEGRO_SAMPLE
Register a handler for al_load_sample_f. The given function will be used to handle the loading of sample files with the given extension.
The extension should include the leading dot (‘.’) character. It will be matched case-insensitively.
The loader
argument may be NULL to unregister an
entry.
Returns true on success, false on error. Returns false if unregistering an entry that doesn’t exist.
See also: al_register_sample_loader
al_register_sample_saver
bool al_register_sample_saver(const char *ext,
bool (*saver)(const char *filename, ALLEGRO_SAMPLE *spl))
Register a handler for al_save_sample. The given function will be used to handle the saving of sample files with the given extension.
The extension should include the leading dot (‘.’) character. It will be matched case-insensitively.
The saver
argument may be NULL to unregister an
entry.
Returns true on success, false on error. Returns false if unregistering an entry that doesn’t exist.
See also: al_register_sample_saver_f, al_register_sample_loader
al_register_sample_saver_f
bool al_register_sample_saver_f(const char *ext,
bool (*saver)(ALLEGRO_FILE* fp, ALLEGRO_SAMPLE *spl))
Register a handler for al_save_sample_f. The given function will be used to handle the saving of sample files with the given extension.
The extension should include the leading dot (‘.’) character. It will be matched case-insensitively.
The saver
argument may be NULL to unregister an
entry.
Returns true on success, false on error. Returns false if unregistering an entry that doesn’t exist.
See also: al_register_sample_saver
al_register_audio_stream_loader
bool al_register_audio_stream_loader(const char *ext,
*(*stream_loader)(const char *filename,
ALLEGRO_AUDIO_STREAM size_t buffer_count, unsigned int samples))
Register a handler for al_load_audio_stream and al_play_audio_stream. The given function will be used to open streams from files with the given extension.
The extension should include the leading dot (‘.’) character. It will be matched case-insensitively.
The stream_loader
argument may be NULL to unregister an
entry.
Returns true on success, false on error. Returns false if unregistering an entry that doesn’t exist.
See also: al_register_audio_stream_loader_f
al_register_audio_stream_loader_f
bool al_register_audio_stream_loader_f(const char *ext,
*(*stream_loader)(ALLEGRO_FILE* fp,
ALLEGRO_AUDIO_STREAM size_t buffer_count, unsigned int samples))
Register a handler for al_load_audio_stream_f and al_play_audio_stream_f. The given function will be used to open streams from files with the given extension.
The extension should include the leading dot (‘.’) character. It will be matched case-insensitively.
The stream_loader
argument may be NULL to unregister an
entry.
Returns true on success, false on error. Returns false if unregistering an entry that doesn’t exist.
See also: al_register_audio_stream_loader
al_register_sample_identifier
bool al_register_sample_identifier(const char *ext,
bool (*identifier)(ALLEGRO_FILE* fp))
Register an identify handler for al_identify_sample. The given function will be used to detect files for the given extension. It will be called with a single argument of type ALLEGRO_FILE which is a file handle opened for reading and located at the first byte of the file. The handler should try to read as few bytes as possible to safely determine if the given file contents correspond to the type with the extension and return true in that case, false otherwise. The file handle must not be closed but there is no need to reset it to the beginning.
The extension should include the leading dot (‘.’) character. It will be matched case-insensitively.
The identifier
argument may be NULL to unregister an
entry.
Returns true on success, false on error. Returns false if unregistering an entry that doesn’t exist.
Since: 5.2.8
See also: al_identify_bitmap
al_identify_sample
char const *al_identify_sample(char const *filename)
This works exactly as al_identify_sample_f but you specify the filename of the file for which to detect the type and not a file handle. The extension, if any, of the passed filename is not taken into account - only the file contents.
Since: 5.2.8
See also: al_init_acodec_addon, al_identify_sample_f, al_register_sample_identifier
al_identify_sample_f
char const *al_identify_sample_f(ALLEGRO_FILE *fp)
Tries to guess the audio file type of the open ALLEGRO_FILE by reading the first few bytes. By default Allegro cannot recognize any file types, but calling al_init_acodec_addon will add detection of the types it can read. You can also use al_register_sample_identifier to add identification for custom file types.
Returns a pointer to a static string with a file extension for the type, including the leading dot. For example “.wav” or “.ogg”. Returns NULL if the audio type cannot be determined.
Since: 5.2.8
See also: al_init_acodec_addon, al_identify_sample, al_register_sample_identifier
Audio recording
Allegro’s audio recording routines give you real-time access to raw, uncompressed audio input streams. Since Allegro hides all of the platform specific implementation details with its own buffering, it will add a small amount of latency. However, for most applications that small overhead will not adversely affect performance.
Recording is supported by the ALSA, AudioQueue, DirectSound8, and PulseAudio drivers. Enumerating or choosing other recording devices is not yet supported.
ALLEGRO_AUDIO_RECORDER
typedef struct ALLEGRO_AUDIO_RECORDER ALLEGRO_AUDIO_RECORDER;
An opaque datatype that represents a recording device.
Since: 5.1.1
Unstable API: The API may need a slight redesign.
Examples:
ALLEGRO_AUDIO_RECORDER_EVENT
typedef struct ALLEGRO_AUDIO_RECORDER_EVENT ALLEGRO_AUDIO_RECORDER_EVENT;
Structure that holds the audio recorder event data. Every event type will contain:
- .source: pointer to the audio recorder
The following will be available depending on the event type:
- .buffer: pointer to buffer containing the audio samples
- .samples: number of samples (not bytes) that are available
Since 5.1.1
See also: al_get_audio_recorder_event
Unstable API: The API may need a slight redesign.
Examples:
al_create_audio_recorder
*al_create_audio_recorder(size_t fragment_count,
ALLEGRO_AUDIO_RECORDER unsigned int samples, unsigned int frequency,
, ALLEGRO_CHANNEL_CONF chan_conf) ALLEGRO_AUDIO_DEPTH depth
Creates an audio recorder using the system’s default recording device. (So if the returned device does not work, try updating the system’s default recording device.)
Allegro will internally buffer several seconds of captured audio with minimal latency. (XXX: These settings need to be exposed via config or API calls.) Audio will be copied out of that private buffer into a fragment buffer of the size specified by the samples parameter. Whenever a new fragment is ready an event will be generated.
The total size of the fragment buffer is fragment_count * samples * bytes_per_sample. It is treated as a circular, never ending buffer. If you do not process the information fast enough, it will be overrun. Because of that, even if you only ever need to process one small fragment at a time, you should still use a large enough value for fragment_count to hold a few seconds of audio.
frequency is the number of samples per second to record. Common values are:
- 8000 - telephone quality speech
- 11025
- 22050
- 44100 - CD quality music (if 16-bit, stereo)
For maximum compatibility, use a depth of ALLEGRO_AUDIO_DEPTH_UINT8 or ALLEGRO_AUDIO_DEPTH_INT16, and a single (mono) channel.
The recorder will not record until you start it with al_start_audio_recorder.
On failure, returns NULL.
Since: 5.1.1
Unstable API: The API may need a slight redesign.
Examples:
al_start_audio_recorder
bool al_start_audio_recorder(ALLEGRO_AUDIO_RECORDER *r)
Begin recording into the fragment buffer. Once a complete fragment has been captured (as specified in al_create_audio_recorder), an ALLEGRO_EVENT_AUDIO_RECORDER_FRAGMENT event will be triggered.
Returns true if it was able to begin recording.
Since: 5.1.1
Unstable API: The API may need a slight redesign.
Examples:
al_stop_audio_recorder
void al_stop_audio_recorder(ALLEGRO_AUDIO_RECORDER *r)
Stop capturing audio data. Note that the audio recorder is still active and consuming resources, so if you are finished recording you should destroy it with al_destroy_audio_recorder.
You may still receive a few events after you call this function as the device flushes the buffer.
If you restart the recorder, it will begin recording at the beginning of the next fragment buffer.
Since: 5.1.1
Unstable API: The API may need a slight redesign.
al_is_audio_recorder_recording
bool al_is_audio_recorder_recording(ALLEGRO_AUDIO_RECORDER *r)
Returns true if the audio recorder is currently capturing data and generating events.
Since: 5.1.1
Unstable API: The API may need a slight redesign.
al_get_audio_recorder_event
*al_get_audio_recorder_event(ALLEGRO_EVENT *event) ALLEGRO_AUDIO_RECORDER_EVENT
Returns the event as an ALLEGRO_AUDIO_RECORDER_EVENT.
Since: 5.1.1
Unstable API: The API may need a slight redesign.
Examples:
al_get_audio_recorder_event_source
*al_get_audio_recorder_event_source(ALLEGRO_AUDIO_RECORDER *r) ALLEGRO_EVENT_SOURCE
Returns the event source for the recorder that generates the various recording events.
Since: 5.1.1
Unstable API: The API may need a slight redesign.
Examples:
al_destroy_audio_recorder
void al_destroy_audio_recorder(ALLEGRO_AUDIO_RECORDER *r)
Destroys the audio recorder and frees all resources associated with it. It is safe to destroy a recorder that is recording.
You may receive events after the recorder has been destroyed. They must be ignored, as the fragment buffer will no longer be valid.
Since: 5.1.1
Unstable API: The API may need a slight redesign.
Examples:
Audio devices
ALLEGRO_AUDIO_DEVICE
typedef struct ALLEGRO_AUDIO_DEVICE ALLEGRO_AUDIO_DEVICE;
An opaque datatype that represents an audio device.
Examples:
al_get_num_audio_output_devices
int al_get_num_audio_output_devices()
Get the number of available audio output devices on the system.
Since: 5.2.8
return -1 for unsupported drivers.
Examples:
al_get_audio_output_device
const ALLEGRO_AUDIO_DEVICE* al_get_audio_output_device(int index)
Get the output audio device of the specified index.
Since: 5.2.8
Examples:
al_get_audio_device_name
const char* al_get_audio_device_name(const ALLEGRO_AUDIO_DEVICE * device)
Get the user friendly display name of the device.
Since: 5.2.8
Examples:
Voices
ALLEGRO_VOICE
typedef struct ALLEGRO_VOICE ALLEGRO_VOICE;
A voice represents an audio device on the system, which may be a real device, or an abstract device provided by the operating system. To play back audio, you would attach a mixer, sample instance or audio stream to a voice.
See also: ALLEGRO_MIXER, ALLEGRO_SAMPLE, ALLEGRO_AUDIO_STREAM
Examples:
al_create_voice
*al_create_voice(unsigned int freq,
ALLEGRO_VOICE , ALLEGRO_CHANNEL_CONF chan_conf) ALLEGRO_AUDIO_DEPTH depth
Creates a voice structure and allocates a voice from the digital sound driver. The passed frequency (in Hz), sample format and channel configuration are used as a hint to what kind of data will be sent to the voice. However, the underlying sound driver is free to use non-matching values. For example, it may be the native format of the sound hardware.
If a mixer is attached to the voice, the mixer will handle the conversion of all its input streams to the voice format and care does not have to be taken for this. However if you access the voice directly, make sure to not rely on the parameters passed to this function, but instead query the returned voice for the actual settings.
Reasonable default arguments are:
(44100, ALLEGRO_AUDIO_DEPTH_INT16, ALLEGRO_CHANNEL_CONF_2) al_create_voice
See also: al_destroy_voice
Examples:
al_destroy_voice
void al_destroy_voice(ALLEGRO_VOICE *voice)
Destroys the voice and deallocates it from the digital driver. Does nothing if the voice is NULL.
See also: al_create_voice
Examples:
al_detach_voice
void al_detach_voice(ALLEGRO_VOICE *voice)
Detaches the mixer, sample instance or audio stream from the voice.
See also: al_attach_mixer_to_voice, al_attach_sample_instance_to_voice, al_attach_audio_stream_to_voice
al_attach_audio_stream_to_voice
bool al_attach_audio_stream_to_voice(ALLEGRO_AUDIO_STREAM *stream,
*voice) ALLEGRO_VOICE
Attaches an audio stream to a voice. The same rules as al_attach_sample_instance_to_voice apply. This may fail if the driver can’t create a voice with the buffer count and buffer size the stream uses.
An audio stream attached directly to a voice has a number of limitations: The audio stream plays immediately and cannot be stopped. The stream position, speed, gain and panning cannot be changed. At this time, we don’t recommend attaching audio streams directly to voices. Use a mixer inbetween.
Returns true on success, false on failure.
See also: al_detach_voice, al_voice_has_attachments
Examples:
al_attach_mixer_to_voice
bool al_attach_mixer_to_voice(ALLEGRO_MIXER *mixer, ALLEGRO_VOICE *voice)
Attaches a mixer to a voice. It must have the same frequency and channel configuration, but the depth may be different.
Returns true on success, false on failure.
See also: al_detach_voice, al_voice_has_attachments
Examples:
al_attach_sample_instance_to_voice
bool al_attach_sample_instance_to_voice(ALLEGRO_SAMPLE_INSTANCE *spl,
*voice) ALLEGRO_VOICE
Attaches a sample instance to a voice, and allows it to play. The instance’s gain and loop mode will be ignored, and it must have the same frequency, channel configuration and depth (including signed-ness) as the voice. This function may fail if the selected driver doesn’t support preloading sample data.
At this time, we don’t recommend attaching sample instances directly to voices. Use a mixer inbetween.
Returns true on success, false on failure.
See also: al_detach_voice, al_voice_has_attachments
Examples:
al_get_voice_frequency
unsigned int al_get_voice_frequency(const ALLEGRO_VOICE *voice)
Return the frequency of the voice (in Hz), e.g. 44100.
al_get_voice_channels
(const ALLEGRO_VOICE *voice) ALLEGRO_CHANNEL_CONF al_get_voice_channels
Return the channel configuration of the voice.
See also: ALLEGRO_CHANNEL_CONF.
al_get_voice_depth
(const ALLEGRO_VOICE *voice) ALLEGRO_AUDIO_DEPTH al_get_voice_depth
Return the audio depth of the voice.
See also: ALLEGRO_AUDIO_DEPTH.
al_get_voice_playing
bool al_get_voice_playing(const ALLEGRO_VOICE *voice)
Return true if the voice is currently playing.
See also: al_set_voice_playing
Examples:
al_set_voice_playing
bool al_set_voice_playing(ALLEGRO_VOICE *voice, bool val)
Change whether a voice is playing or not. This can only work if the voice has a non-streaming object attached to it, e.g. a sample instance. On success the voice’s current sample position is reset.
Returns true on success, false on failure.
See also: al_get_voice_playing
Examples:
al_get_voice_position
unsigned int al_get_voice_position(const ALLEGRO_VOICE *voice)
When the voice has a non-streaming object attached to it, e.g. a sample, returns the voice’s current sample position. Otherwise, returns zero.
See also: al_set_voice_position.
al_set_voice_position
bool al_set_voice_position(ALLEGRO_VOICE *voice, unsigned int val)
Set the voice position. This can only work if the voice has a non-streaming object attached to it, e.g. a sample instance.
Returns true on success, false on failure.
See also: al_get_voice_position.
al_voice_has_attachments
bool al_voice_has_attachments(const ALLEGRO_VOICE* voice)
Returns true if the voice has something attached to it.
See also: al_attach_mixer_to_voice, al_attach_sample_instance_to_voice, al_attach_audio_stream_to_voice
Since: 5.2.9
Mixers
ALLEGRO_MIXER
typedef struct ALLEGRO_MIXER ALLEGRO_MIXER;
A mixer mixes together attached streams into a single buffer. In the process, it converts channel configurations, sample frequencies and audio depths of the attached sample instances and audio streams accordingly. You can control the quality of this conversion using ALLEGRO_MIXER_QUALITY.
When going from mono to stereo (and above), the mixer reduces the
volume of both channels by sqrt(2)
. When going from stereo
(and above) to mono, the mixer reduces the volume of the left and right
channels by sqrt(2)
before adding them to the center
channel (if present).
Examples:
ALLEGRO_MIXER_QUALITY
enum ALLEGRO_MIXER_QUALITY
- ALLEGRO_MIXER_QUALITY_POINT - point sampling
- ALLEGRO_MIXER_QUALITY_LINEAR - linear interpolation
- ALLEGRO_MIXER_QUALITY_CUBIC - cubic interpolation (since: 5.0.8, 5.1.4)
al_create_mixer
*al_create_mixer(unsigned int freq,
ALLEGRO_MIXER , ALLEGRO_CHANNEL_CONF chan_conf) ALLEGRO_AUDIO_DEPTH depth
Creates a mixer to attach sample instances, audio streams, or other mixers to. It will mix into a buffer at the requested frequency (in Hz) and channel count.
The only supported audio depths are ALLEGRO_AUDIO_DEPTH_FLOAT32 and ALLEGRO_AUDIO_DEPTH_INT16 (not yet complete).
To actually produce any output, the mixer will have to be attached to a voice using al_attach_mixer_to_voice.
Reasonable default arguments are:
(44100, ALLEGRO_AUDIO_DEPTH_FLOAT32, ALLEGRO_CHANNEL_CONF_2) al_create_mixer
Returns true on success, false on error.
See also: al_destroy_mixer, ALLEGRO_AUDIO_DEPTH, ALLEGRO_CHANNEL_CONF
Examples:
al_destroy_mixer
void al_destroy_mixer(ALLEGRO_MIXER *mixer)
Destroys the mixer.
See also: al_create_mixer
Examples:
al_get_default_mixer
*al_get_default_mixer(void) ALLEGRO_MIXER
Return the default mixer, or NULL if one has not been set. Although different configurations of mixers and voices can be used, in most cases a single mixer attached to a voice is what you want. The default mixer is used by al_play_sample.
See also: al_reserve_samples, al_play_sample, al_set_default_mixer, al_restore_default_mixer
Examples:
al_set_default_mixer
bool al_set_default_mixer(ALLEGRO_MIXER *mixer)
Sets the default mixer. All samples started with al_play_sample will be stopped and all sample instances returned by al_lock_sample_id will be invalidated. If you are using your own mixer, this should be called before al_reserve_samples.
Returns true on success, false on error.
See also: al_reserve_samples, al_play_sample, al_get_default_mixer, al_restore_default_mixer
al_restore_default_mixer
bool al_restore_default_mixer(void)
Restores Allegro’s default mixer and attaches it to the default voice. If the default mixer hasn’t been created before, it will be created. If the default voice hasn’t been set via al_set_default_voice or created before, it will also be created. All samples started with al_play_sample will be stopped and all sample instances returned by al_lock_sample_id will be invalidated.
Returns true on success, false on error.
See also: al_get_default_mixer, al_set_default_mixer, al_reserve_samples.
al_get_default_voice
*al_get_default_voice(void) ALLEGRO_VOICE
Returns the default voice or NULL if there is none.
Since: 5.1.13
See also: al_get_default_mixer
al_set_default_voice
void al_set_default_voice(ALLEGRO_VOICE *voice)
You can call this before calling al_restore_default_mixer to provide the voice which should be used. Any previous voice will be destroyed. You can also pass NULL to destroy the current default voice.
Since: 5.1.13
See also: al_get_default_mixer
al_attach_mixer_to_mixer
bool al_attach_mixer_to_mixer(ALLEGRO_MIXER *stream, ALLEGRO_MIXER *mixer)
Attaches the mixer passed as the first argument onto the mixer passed as the second argument. The first mixer (that is going to be attached) must not already be attached to anything. Both mixers must use the same frequency, audio depth and channel configuration.
Returns true on success, false on error.
It is invalid to attach a mixer to itself.
See also: al_detach_mixer.
Examples:
al_attach_sample_instance_to_mixer
bool al_attach_sample_instance_to_mixer(ALLEGRO_SAMPLE_INSTANCE *spl,
*mixer) ALLEGRO_MIXER
Attach a sample instance to a mixer. The instance must not already be attached to anything.
Returns true on success, false on failure.
See also: al_detach_sample_instance.
Examples:
al_attach_audio_stream_to_mixer
bool al_attach_audio_stream_to_mixer(ALLEGRO_AUDIO_STREAM *stream, ALLEGRO_MIXER *mixer)
Attach an audio stream to a mixer. The stream must not already be attached to anything.
Returns true on success, false on failure.
See also: al_detach_audio_stream.
Examples:
al_get_mixer_frequency
unsigned int al_get_mixer_frequency(const ALLEGRO_MIXER *mixer)
Return the mixer frequency (in Hz).
See also: al_set_mixer_frequency
al_set_mixer_frequency
bool al_set_mixer_frequency(ALLEGRO_MIXER *mixer, unsigned int val)
Set the mixer frequency (in Hz). This will only work if the mixer is not attached to anything.
Returns true on success, false on failure.
See also: al_get_mixer_frequency
al_get_mixer_channels
(const ALLEGRO_MIXER *mixer) ALLEGRO_CHANNEL_CONF al_get_mixer_channels
Return the mixer channel configuration.
See also: ALLEGRO_CHANNEL_CONF.
Examples:
al_get_mixer_depth
(const ALLEGRO_MIXER *mixer) ALLEGRO_AUDIO_DEPTH al_get_mixer_depth
Return the mixer audio depth.
See also: ALLEGRO_AUDIO_DEPTH.
Examples:
al_get_mixer_gain
float al_get_mixer_gain(const ALLEGRO_MIXER *mixer)
Return the mixer gain (amplification factor). The default is 1.0.
Since: 5.0.6, 5.1.0
See also: al_set_mixer_gain.
Examples:
al_set_mixer_gain
bool al_set_mixer_gain(ALLEGRO_MIXER *mixer, float new_gain)
Set the mixer gain (amplification factor).
Returns true on success, false on failure.
Since: 5.0.6, 5.1.0
See also: al_get_mixer_gain
Examples:
al_get_mixer_quality
(const ALLEGRO_MIXER *mixer) ALLEGRO_MIXER_QUALITY al_get_mixer_quality
Return the mixer quality.
See also: ALLEGRO_MIXER_QUALITY, al_set_mixer_quality
al_set_mixer_quality
bool al_set_mixer_quality(ALLEGRO_MIXER *mixer, ALLEGRO_MIXER_QUALITY new_quality)
Set the mixer quality. This can only succeed if the mixer does not have anything attached to it.
Returns true on success, false on failure.
See also: ALLEGRO_MIXER_QUALITY, al_get_mixer_quality
al_get_mixer_playing
bool al_get_mixer_playing(const ALLEGRO_MIXER *mixer)
Return true if the mixer is playing.
See also: al_set_mixer_playing.
Examples:
al_set_mixer_playing
bool al_set_mixer_playing(ALLEGRO_MIXER *mixer, bool val)
Change whether the mixer is playing.
Returns true on success, false on failure.
See also: al_get_mixer_playing.
Examples:
al_get_mixer_attached
bool al_get_mixer_attached(const ALLEGRO_MIXER *mixer)
Return true if the mixer is attached to something.
See also: al_mixer_has_attachments, al_attach_sample_instance_to_mixer, al_attach_audio_stream_to_mixer, al_attach_mixer_to_mixer, al_detach_mixer
al_mixer_has_attachments
bool al_mixer_has_attachments(const ALLEGRO_MIXER* mixer)
Returns true if the mixer has something attached to it.
See also: al_get_mixer_attached, al_attach_sample_instance_to_mixer, al_attach_audio_stream_to_mixer, al_attach_mixer_to_mixer, al_detach_mixer
Since: 5.2.9
al_detach_mixer
bool al_detach_mixer(ALLEGRO_MIXER *mixer)
Detach the mixer from whatever it is attached to, if anything.
See also: al_attach_mixer_to_mixer.
Examples:
al_set_mixer_postprocess_callback
bool al_set_mixer_postprocess_callback(ALLEGRO_MIXER *mixer,
void (*pp_callback)(void *buf, unsigned int samples, void *data),
void *pp_callback_userdata)
Sets a post-processing filter function that’s called after the attached streams have been mixed. The buffer’s format will be whatever the mixer was created with. The sample count and user-data pointer is also passed.
Note: The callback is called from a dedicated audio thread.
Examples:
Miscelaneous
ALLEGRO_AUDIO_DEPTH
enum ALLEGRO_AUDIO_DEPTH
Sample depth and type as well as signedness. Mixers only use 32-bit signed float (-1..+1), or 16-bit signed integers. Signedness is determined by an “unsigned” bit-flag applied to the depth value.
- ALLEGRO_AUDIO_DEPTH_INT8
- ALLEGRO_AUDIO_DEPTH_INT16
- ALLEGRO_AUDIO_DEPTH_INT24
- ALLEGRO_AUDIO_DEPTH_FLOAT32
- ALLEGRO_AUDIO_DEPTH_UNSIGNED
For convenience:
- ALLEGRO_AUDIO_DEPTH_UINT8
- ALLEGRO_AUDIO_DEPTH_UINT16
- ALLEGRO_AUDIO_DEPTH_UINT24
Examples:
ALLEGRO_AUDIO_PAN_NONE
#define ALLEGRO_AUDIO_PAN_NONE (-1000.0f)
A special value for the pan property of sample instances and audio streams. Use this value to disable panning on sample instances and audio streams, and play them without attentuation implied by panning support.
ALLEGRO_AUDIO_PAN_NONE is different from a pan value of 0.0 (centered) because, when panning is enabled, we try to maintain a constant sound power level as a sample is panned from left to right. A sound coming out of one speaker should sound as loud as it does when split over two speakers. As a consequence, a sample with pan value 0.0 will be 3 dB softer than the original level.
(Please correct us if this is wrong.)
Examples:
ALLEGRO_CHANNEL_CONF
enum ALLEGRO_CHANNEL_CONF
Speaker configuration (mono, stereo, 2.1, etc).
- ALLEGRO_CHANNEL_CONF_1
- ALLEGRO_CHANNEL_CONF_2
- ALLEGRO_CHANNEL_CONF_3
- ALLEGRO_CHANNEL_CONF_4
- ALLEGRO_CHANNEL_CONF_5_1
- ALLEGRO_CHANNEL_CONF_6_1
- ALLEGRO_CHANNEL_CONF_7_1
Examples:
ALLEGRO_PLAYMODE
enum ALLEGRO_PLAYMODE
Sample and stream playback mode.
- ALLEGRO_PLAYMODE_ONCE - the sample/stream is played from start to finish an then it stops.
- ALLEGRO_PLAYMODE_LOOP - the sample/stream is played from start to finish (or between the two loop points). When it reaches the end, it restarts from the beginning.
- ALLEGRO_PLAYMODE_LOOP_ONCE - just like ALLEGRO_PLAYMODE_ONCE, but respects the loop end point.
- ALLEGRO_PLAYMODE_BIDIR - the sample is played from start to finish (or between the two loop points). When it reaches the end, it reverses the playback direction and plays until it reaches the beginning when it reverses the direction back to normal. This is mode is rarely supported for streams.
Examples:
ALLEGRO_AUDIO_EVENT_TYPE
enum ALLEGRO_AUDIO_EVENT_TYPE
Events sent by al_get_audio_stream_event_source or al_get_audio_recorder_event_source.
ALLEGRO_EVENT_AUDIO_STREAM_FRAGMENT
Sent when a stream fragment is ready to be filled in. See al_get_audio_stream_fragment.
ALLEGRO_EVENT_AUDIO_STREAM_FINISHED
Sent when a stream is finished.
ALLEGRO_EVENT_AUDIO_RECORDER_FRAGMENT
Sent after a user-specified number of samples have been recorded. Convert this to ALLEGRO_AUDIO_RECORDER_EVENT via al_get_audio_recorder_event.
You must always check the values for the buffer and samples as they are not guaranteed to be exactly what was originally specified.
Since: 5.1.1
Unstable API: The API may need a slight redesign.
al_get_allegro_audio_version
uint32_t al_get_allegro_audio_version(void)
Returns the (compiled) version of the addon, in the same format as al_get_allegro_version.
al_get_audio_depth_size
size_t al_get_audio_depth_size(ALLEGRO_AUDIO_DEPTH depth)
Return the size of a sample, in bytes, for the given format. The format is one of the values listed under ALLEGRO_AUDIO_DEPTH.
Examples:
al_get_channel_count
size_t al_get_channel_count(ALLEGRO_CHANNEL_CONF conf)
Return the number of channels for the given channel configuration, which is one of the values listed under ALLEGRO_CHANNEL_CONF.
Examples:
al_fill_silence
void al_fill_silence(void *buf, unsigned int samples,
, ALLEGRO_CHANNEL_CONF chan_conf) ALLEGRO_AUDIO_DEPTH depth
Fill a buffer with silence, for the given format and channel configuration. The buffer must have enough space for the given number of samples, and be properly aligned.
Since: 5.1.8
Examples: